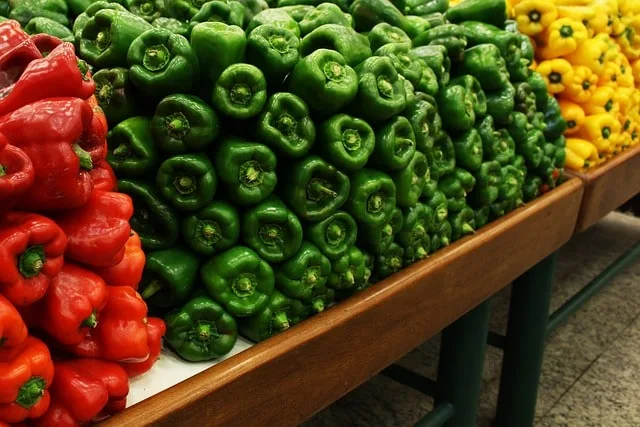
Unraveling KNN in Machine Learning
one of the machine learning algorithms that has piqued my interest recently is K-Nearest Neighbors, or KNN for short.
Furthermore, in this article, I’ll share my insights into KNN, its applications, and how it works. So, let’s get started!
What is KNN in Machine Learning?
KNN is a simple yet powerful supervised learning algorithm we can use for classification and regression tasks.
Moreover, the main idea behind KNN is that similar data points tend to be closer together in feature space.
Why Do We Use KNN in Machine Learning?
It’s popular in machine learning because it:
- Is easy to understand and implement
- Adapts well to new data
- Performs well with small datasets
- Requires minimal training time
- Is a non-parametric method, making no assumptions about the data distribution
KNN Algorithm: A Machine Learning Workhorse
KNN algorithm operates by finding the k nearest neighbors of a new data point and determining its class or value based on a majority vote or averaging.
It’s particularly useful in solving problems where:
- The data is noisy
- The decision boundary is irregular
- The relationships between features are complex
KNN Algorithm Example: A Quick Illustration
Imagine we have a dataset containing information about fruits, with features like color, size, and texture.
In order to classify an unknown fruit, we can use KNN by finding its k nearest neighbors in the dataset and assigning the most common fruit label among them.
Example with code
Here’s an example of how to implement the KNN algorithm using Python and the sklearn library.
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import accuracy_score
# Load the Iris dataset
iris = load_iris()
X = iris.data
y = iris.target
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Normalize the data
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
# Create the KNN classifier
k = 3
knn = KNeighborsClassifier(n_neighbors=k)
# Train the classifier
knn.fit(X_train, y_train)
# Make predictions on the test set
y_pred = knn.predict(X_test)
# Calculate the accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f"KNN accuracy: {accuracy:.2f}")
This code demonstrates how to use the KNN algorithm with sklearn library to classify the famous Iris dataset.
In order to train our KNN classifier model, we need to split the data into training and testing sets. After that we also need to normalize it.
Finally, we train the KNN classifier and evaluate it.
What is KNN Best Suited for?
KNN excels in scenarios where:
- The dataset is small to moderately-sized
- The decision boundaries are irregular
- The problem involves multi-class classification or regression
However, it might struggle with large datasets and high-dimensional feature spaces.
KNN in Real Life: Practical Applications
KNN has a wide range of real-life applications, such as:
- Recommender systems
- Image recognition
- Handwriting analysis
- Medical diagnosis
- Anomaly detection
How Does KNN Work Step by Step?
To implement KNN, follow these steps:
- Choose the number of neighbors k and the distance metric.
- Calculate the distance between the new data point and all training data points.
- Select the k nearest neighbors.
- Determine the class or value of the new data point based on a majority vote or averaging.
What Distance is Used in KNN?
Various distance metrics can be used in KNN, including:
- Euclidean distance: The straight-line distance between two points
- Manhattan distance: The sum of the absolute differences between coordinates
- Minkowski distance: A generalized distance metric that encompasses Euclidean and Manhattan distances
Moreover, the choice of distance metric depends on the problem and the nature of the data.
Conclusion
In conclusion, KNN is a versatile and intuitive algorithm that plays a significant role in machine learning.
Furthermore, its simplicity, adaptability, and effectiveness make it a popular choice for solving diverse problems in classification and regression.