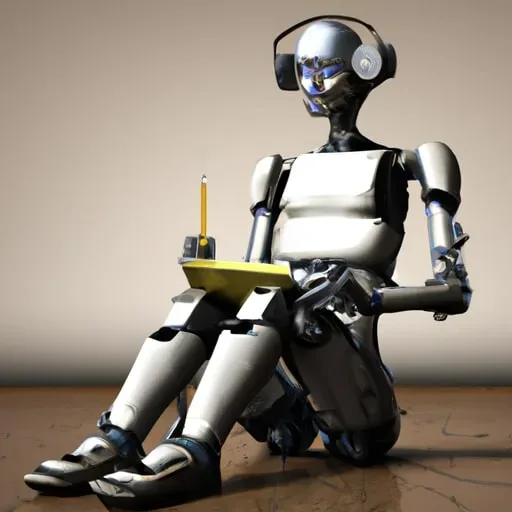
How To Use Image Generator From OpenAI With Python
In this post, we’re going to make a simple python script, which will use DALL-E image generator model from OpenAI. Additionally, we’re going to use the OpenAIs API, which you can only use if you upgraded for paid plan.
Luckily, the API calls aren’t really expensive and you can also use other machine learning models from OpenAI with it.
But before, we get to coding, let’s talk about what DALL-E model is, and how does it work. In essence, it’s a deep learning model that can generate images from natural language descriptions.
Prerequisites
Okay, before we start coding, you’ll need to create an API key on OpenAI website. Furthermore, we’re going to store this key in a separate file, so we don’t reveal it in the script.
First, let’s import all the necessary libraries and tools, that we’ll need for this project.
import os
import json
import openai
import urllib.request
from urllib.parse import urlparse
Okay, now let’s write code for the function that will fetch our API key from auth.json file. Keep in mind that I created this file in the same directory as the script. In case you want to store it in a separate folder, you’ll also need to update the paths in code.
ROOT = os.path.dirname(__file__)
def get_token(token_name):
auth_file = open(os.path.join(ROOT, 'auth.json'))
auth_data = json.load(auth_file)
token = auth_data[token_name]
return token
openai.api_key = get_token('openai-token')
Great! Now, we’re ready to start working with DALL-E model.
Getting response from OpenAI image generator model
Since the model needs text input, we need to set our prompt, which we’ll pass into the generator. Next, we’ll call a function that will prompt OpenAIs model to start generating and give us the response in a for of an url address that leads to the generated image.
And for the last step, we’ll take that url and download the image locally and give it the same name as the generator model did. This way, we can be sure that consecutive calls won’t save over existing images.
prompt = 'Futuristic robot artist'
response = openai.Image.create(
prompt=prompt,
n=1,
size='512x512'
)
image_url = response['data'][0]['url']
parsed_url = urlparse(image_url)
image_path = os.path.join(ROOT, os.path.basename(parsed_url.path))
urllib.request.urlretrieve(image_url, image_path)
That’s it! Following image is what we got from the prompt we provided.
Conclusion
To conclude, we made a simple script that uses OpenAI image generator model DALL-E. I learned a lot while working on this project and I hope it proves helpful to you as well.