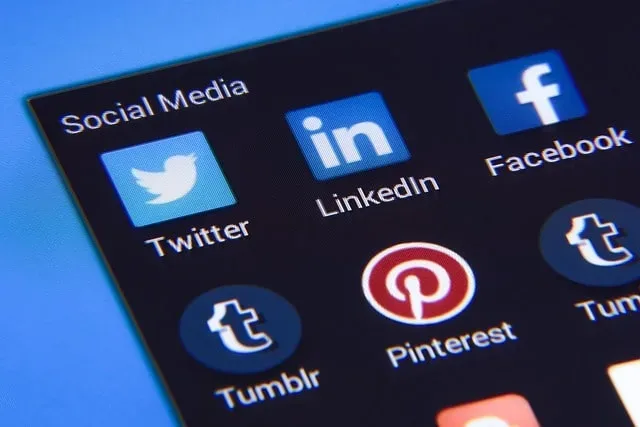
How To Authorize Tumblr API With OAuth 1 Protocol
In this post, we’re going to take a look at the authorization process for Tumblr API use. In case you’re already familiar with authorization protocols, we’ll demonstrate how to use OAuth 1 protocol.
Usually, when we’re trying to use any kind of API, the provider gives us everything we need so it’s all plug and play. However, this is not the case with Tumblr API. The purpose of this tutorial is to show you how to get over this authentication hurdle.
Setting up Tumblr API application
First of all, we need to register our application on Tumblr, which also means that you’ll need a Tumblr account. Furthermore, you can register with your Google account, which makes it very easy, since all you need to do is click a button.
When you’re ready to register your application, you’ll need to input its name, description, and redirect website. Additionally, it needs an email address, but Tumblr takes care of this by default by using your Google account email.
You may be asking yourself, why do I need a website for this. It’s because the authorization process will lead you to a link where you’ll need to allow privilages to your application. And after you click allow, it will send you to the website you provide, with several attributes inside the URL that you’ll need to get the access token data.
Getting all necessary tokens
Once you register your application, you’ll be able to get the consumer key and secret from it on Tumblr. However, that’s only half of all the tokens you’ll need to make this thing work. Further, we’ll take that consumer key and secret and save it inside auth.json file.
But before we get to the part where we’ll need them, let’s just define variables with URLs we’ll need for the authorization. Additionally, you can find these URLs below the button for registering new application.
request_token_url = 'http://www.tumblr.com/oauth/request_token'
authorize_url = 'http://www.tumblr.com/oauth/authorize'
access_token_url = 'http://www.tumblr.com/oauth/access_token'
Next, we’ll fetch the consumer key and secret from the json file we mentioned before.
with open(os.path.join(ROOT, 'auth.json'), 'r') as auth_file:
auth_data = json.load(auth_file)
consumer_key = auth_data['tumblr-tokens']['consumer-key']
consumer_secret = auth_data['tumblr-tokens']['consumer-secret']
In the following step, we’ll need to go to the link that’ll lead us to the privilages confirmation page. As we mentioned before, after clicking on Allow button, it will send us to the redirect URL.
Furthermore, we’ll only have to do this once for each application. Therefore, we’ll save this URL inside auth.json file to check whether it’s the first time we’re getting our access token or not.
oauth_session = OAuth1Session(consumer_key, client_secret=consumer_secret)
fetch_response = oauth_session.fetch_request_token(request_token_url)
resource_owner_key = fetch_response.get('oauth_token')
resource_owner_secret = fetch_response.get('oauth_token_secret')
full_authorize_url = oauth_session.authorization_url(authorize_url)
if 'redirect-response' not in auth_data['tumblr-tokens']:
print(f'\nPlease go here and authorize: \n{full_authorize_url}')
redirect_response = input('Allow then paste the full redirect URL here:\n').strip()
auth_data['tumblr-tokens']['redirect-response'] = redirect_response
oauth_response = oauth_session.parse_authorization_response(redirect_response)
verifier = oauth_response.get('oauth_verifier')
oauth_session = OAuth1Session(
consumer_key,
client_secret=consumer_secret,
resource_owner_key=resource_owner_key,
resource_owner_secret=resource_owner_secret,
verifier=verifier
)
oauth_tokens = oauth_session.fetch_access_token(access_token_url)
with open(os.path.join(ROOT, 'auth.json'), 'w') as auth_file:
auth_data['tumblr-tokens']['oauth-token'] = oauth_tokens.get('oauth_token')
auth_data['tumblr-tokens']['oauth-token-secret'] = oauth_tokens.get('oauth_token_secret')
json.dump(auth_data, auth_file, indent=4)
oauth_token = auth_data['tumblr-tokens']['oauth-token']
oauth_token_secret = auth_data['tumblr-tokens']['oauth-token-secret']
else:
oauth_token = auth_data['tumblr-tokens']['oauth-token']
oauth_token_secret = auth_data['tumblr-tokens']['oauth-token-secret']
So, as you can see, there’s quite a bit going on in this process. But don’t worry, majority of this code will only fire once, when we’re first gaining access to the OAuth token and secret. After we get them, we save them to json file for future use.
Even more, let’s put all this logic into a function, which we’ll call to get all our tokens for getting access to Tumblr API.
def get_tokens():
request_token_url = 'http://www.tumblr.com/oauth/request_token'
authorize_url = 'http://www.tumblr.com/oauth/authorize'
access_token_url = 'http://www.tumblr.com/oauth/access_token'
with open(os.path.join(ROOT, 'auth.json'), 'r') as auth_file:
auth_data = json.load(auth_file)
consumer_key = auth_data['tumblr-tokens']['consumer-key']
consumer_secret = auth_data['tumblr-tokens']['consumer-secret']
oauth_session = OAuth1Session(consumer_key, client_secret=consumer_secret)
fetch_response = oauth_session.fetch_request_token(request_token_url)
resource_owner_key = fetch_response.get('oauth_token')
resource_owner_secret = fetch_response.get('oauth_token_secret')
full_authorize_url = oauth_session.authorization_url(authorize_url)
if 'redirect-response' not in auth_data['tumblr-tokens']:
print(f'\nPlease go here and authorize: \n{full_authorize_url}')
redirect_response = input('Allow then paste the full redirect URL here:\n').strip()
auth_data['tumblr-tokens']['redirect-response'] = redirect_response
oauth_response = oauth_session.parse_authorization_response(redirect_response)
verifier = oauth_response.get('oauth_verifier')
oauth_session = OAuth1Session(
consumer_key,
client_secret=consumer_secret,
resource_owner_key=resource_owner_key,
resource_owner_secret=resource_owner_secret,
verifier=verifier
)
oauth_tokens = oauth_session.fetch_access_token(access_token_url)
with open(os.path.join(ROOT, 'auth.json'), 'w') as auth_file:
auth_data['tumblr-tokens']['oauth-token'] = oauth_tokens.get('oauth_token')
auth_data['tumblr-tokens']['oauth-token-secret'] = oauth_tokens.get('oauth_token_secret')
json.dump(auth_data, auth_file, indent=4)
oauth_token = auth_data['tumblr-tokens']['oauth-token']
oauth_token_secret = auth_data['tumblr-tokens']['oauth-token-secret']
else:
oauth_token = auth_data['tumblr-tokens']['oauth-token']
oauth_token_secret = auth_data['tumblr-tokens']['oauth-token-secret']
return {
'consumer_key': consumer_key,
'consumer_secret': consumer_secret,
'oauth_token': oauth_token,
'oauth_token_secret': oauth_token_secret
}
Now, we’re finally ready to use the API!
tokens = get_tokens()
client = pytumblr2.TumblrRestClient(
tokens['consumer_key'],
tokens['consumer_secret'],
tokens['oauth_token'],
tokens['oauth_token_secret']
)
print(client.info())
Conclusion
To conclude, we made a function that handles authorization process for Tumblr API applications. Furthermore, this will make working with Tumblr API a simple plug and play experience.
In addition, I learned a lot while working on this project and I hope this will be helpful for you as well. If you liked this tutorial, I recommend you check out my other python projects including AI and Discord API.