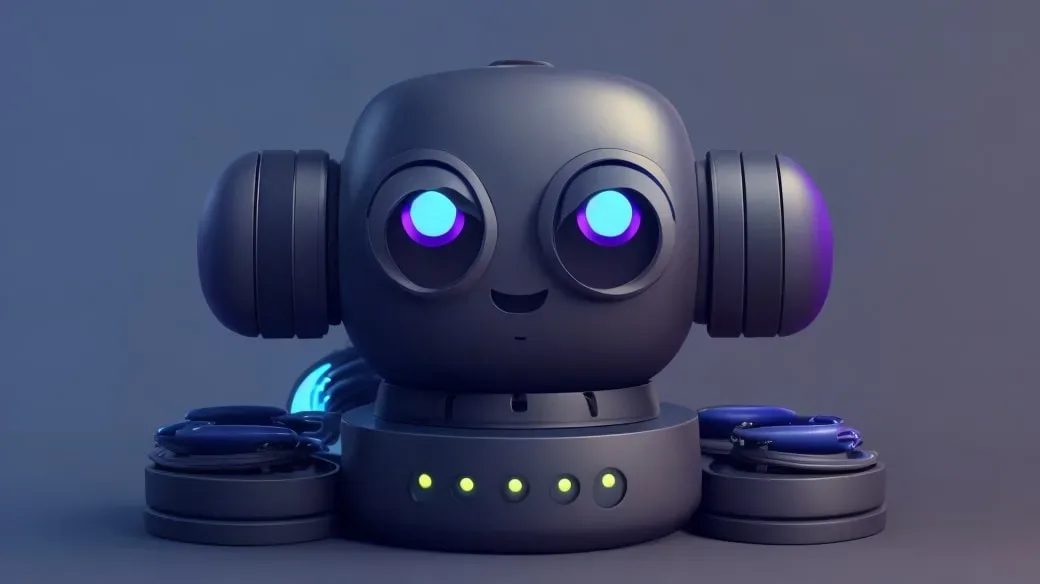
How To Add Buttons To Your Discord Bot With Python
In this post, we’re going to explore Discord API functionalities, that allow us to add buttons to our bots. Additionally, we’ll check out various different predefined colors, we can use for them.
We can use these buttons for just about anything, because we can trigger functions with them. However, we’ll focus more on the estetic part and just make the bot reply back with a short message.
Setup
In case this is your first time working on a Discord bot, you’ll need to ste a couple of things up before you get coding.
Firstly, you need to create an application on Discord Developer Portal and get the access token for your bot.
Secondly, you need to get your bot into your Discord server, which you can do via a generated URL. Furthermore, you can find this URL generator under OAuth2 tab under your apps settings. In order to generate your URL, you need to select scope (bot) and bot permissions.
And with that, you’re now ready to start coding your Discord bot.
Coding Discord bot with buttons
Alright, first things first, let’s set our access token as a environmental variable. We can do this by saving it in the “.env” file (DISCORD_TOKEN=input-your-token). In case you’re going to push this project on a public repository on GitHub, you should also add this file to the “.gitignore” file.
Next, let’s import all necessary libraries and tools that we’ll need.
import os
from dotenv import load_dotenv
import discord
from discord.ext import commands
Alright, now let’s get the value of our access token and store it in a variable.
load_dotenv()
DISCORD_TOKEN = os.getenv("DISCORD_TOKEN")
The following snippet is the bare bones code you need to get the Discord bot running. It includes creating a bot instance, defining the on_ready event and one command. Furthermore, we’re going to use this command to make the bot post buttons in chat.
def run():
intents = discord.Intents.default()
intents.message_content = True
bot = commands.Bot(
command_prefix=commands.when_mentioned_or('!'),
description='Buttons demo',
intents=intents
)
@bot.event
async def on_ready():
print(f'Logged in as {bot.user} (ID: {bot.user.id})')
print('------')
await bot.tree.sync()
@bot.hybrid_command(name='buttons')
async def buttons(ctx):
view = CustomView()
message = await ctx.send(view=view)
view.message = message
await view.wait()
bot.run(DISCORD_TOKEN)
if __name__ == "__main__":
run()
We’re also need to define a class for CustomView, where we define all of the buttons that will appear in it.
class CustomView(discord.ui.View):
@discord.ui.button(label='blurple', style=discord.ButtonStyle.blurple)
async def blurple(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on blurple button.')
@discord.ui.button(label='gray', style=discord.ButtonStyle.gray)
async def gray(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on gray button.')
@discord.ui.button(label='grey', style=discord.ButtonStyle.grey)
async def grey(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on grey button.')
@discord.ui.button(label='green', style=discord.ButtonStyle.green)
async def green(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on green button.')
@discord.ui.button(label='red', style=discord.ButtonStyle.red)
async def red(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on red button.')
@discord.ui.button(label='primary', style=discord.ButtonStyle.primary)
async def primary(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on primary button.')
@discord.ui.button(label='secondary', style=discord.ButtonStyle.secondary)
async def secondary(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on secondary button.')
@discord.ui.button(label='success', style=discord.ButtonStyle.success)
async def success(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on success button.')
@discord.ui.button(label='danger', style=discord.ButtonStyle.danger)
async def danger(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on danger button.')
That’s it! Now you can run the bot and press away at brand new buttons in your Discord chat.
Here is also the entire code of this project.
import os
from dotenv import load_dotenv
import discord
from discord.ext import commands
load_dotenv()
DISCORD_TOKEN = os.getenv("DISCORD_TOKEN")
class CustomView(discord.ui.View):
@discord.ui.button(label='blurple', style=discord.ButtonStyle.blurple)
async def blurple(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on blurple button.')
@discord.ui.button(label='gray', style=discord.ButtonStyle.gray)
async def gray(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on gray button.')
@discord.ui.button(label='grey', style=discord.ButtonStyle.grey)
async def grey(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on grey button.')
@discord.ui.button(label='green', style=discord.ButtonStyle.green)
async def green(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on green button.')
@discord.ui.button(label='red', style=discord.ButtonStyle.red)
async def red(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on red button.')
@discord.ui.button(label='primary', style=discord.ButtonStyle.primary)
async def primary(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on primary button.')
@discord.ui.button(label='secondary', style=discord.ButtonStyle.secondary)
async def secondary(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on secondary button.')
@discord.ui.button(label='success', style=discord.ButtonStyle.success)
async def success(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on success button.')
@discord.ui.button(label='danger', style=discord.ButtonStyle.danger)
async def danger(self, interaction: discord.Interaction, button: discord.ui.Button):
await interaction.response.send_message('You just click on danger button.')
def run():
intents = discord.Intents.default()
intents.message_content = True
bot = commands.Bot(
command_prefix=commands.when_mentioned_or('!'),
description='Buttons demo',
intents=intents
)
@bot.event
async def on_ready():
print(f'Logged in as {bot.user} (ID: {bot.user.id})')
print('------')
await bot.tree.sync()
@bot.hybrid_command(name='buttons')
async def buttons(ctx):
view = CustomView()
message = await ctx.send(view=view)
view.message = message
await view.wait()
bot.run(DISCORD_TOKEN)
if __name__ == "__main__":
run()
Conclusion
To conclude, we made a simple Discord bot to demonstrate how to create buttons and display them in chat. I learned a lot while working on this project and I hope you will find it helpful as well.