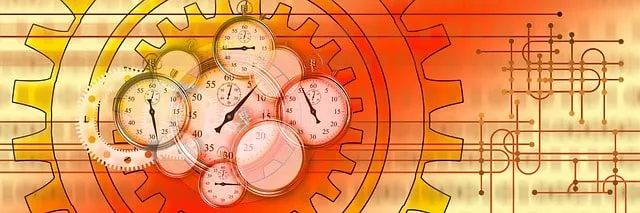
Python Bayesian Optimization in ML
Bayesian optimization is a cutting-edge technique in machine learning for hyperparameter tuning. Furthermore, we’re also going to demonstrate how it works using python.
By smartly selecting the best parameter values, it optimizes model performance. Unlike traditional methods, it relies on probabilistic models to make informed decisions, often yielding faster and more accurate results.
Hyperparameter tuning plays a vital role in the machine learning process. As it enhances a model’s accuracy and efficiency by finding the optimal parameter combination.
These parameters, not learned during training, control various aspects of the model’s architecture and learning.
How to Perform Bayesian Optimization in Python
Essential Libraries and Tools
To implement Bayesian optimization in Python, you’ll need the following libraries and tools:
- Scikit-learn: A popular machine learning library that provides various tools, including those for model evaluation.
- Scikit-optimize: A library specifically designed for optimization tasks, including Bayesian optimization.
Step-by-Step Guide
Follow these steps to perform Bayesian optimization in Python:
- Import necessary libraries: Start by importing scikit-learn, scikit-optimize, and other required libraries.
- Define your model: Choose the machine learning algorithm you’d like to optimize and set it up using scikit-learn.
- Specify the search space: Identify the hyperparameters you want to tune and their respective ranges.
- Select a surrogate model: Pick a probabilistic model, such as Gaussian Processes, to approximate the objective function.
- Create an acquisition function: Choose a suitable acquisition function to guide the search for optimal hyperparameters.
- Initialize with random samples: Start by evaluating a few random samples from the search space to initialize the Bayesian optimization process.
- Update the surrogate model: Use the results of the sampled points to update the surrogate model.
- Choose the next sample: Apply the acquisition function to the surrogate model to identify the most promising point in the search space to sample next.
- Iterate and optimize: Repeat steps 7 and 8 until reaching a stopping criterion.
- Evaluate the best hyperparameters: Use the optimized hyperparameters in your machine learning model and assess its performance.
By following these steps, you can effectively leverage Bayesian optimization in Python to find the optimal hyperparameters.
import numpy as np
from sklearn.datasets import make_classification
from sklearn.model_selection import cross_val_score
from sklearn.ensemble import RandomForestClassifier
from skopt import BayesSearchCV
from skopt.space import Real, Categorical, Integer
# Step 1: Import necessary libraries
# Step 2: Define your model
model = RandomForestClassifier()
# Step 3: Specify the search space
param_space = {
"n_estimators": Integer(10, 200),
"max_depth": Integer(1, 50),
"min_samples_split": Real(0.001, 1.0),
"min_samples_leaf": Integer(1, 50),
"criterion": Categorical(["gini", "entropy"]),
}
# Step 4-5: Select a surrogate model and create an acquisition function
# Scikit-optimize's BayesSearchCV does this automatically
# Step 6-10: Initialize with random samples, update the surrogate model, choose the next sample, and iterate
bayes_search = BayesSearchCV(
model,
param_space,
n_iter=50,
cv=5,
n_jobs=-1,
scoring="accuracy",
random_state=42,
)
# Load sample dataset and split it into features (X) and target (y)
X, y = make_classification(n_samples=1000, n_features=20, n_classes=2, random_state=42)
# Perform Bayesian optimization
bayes_search.fit(X, y)
# Retrieve the best hyperparameters
best_params = bayes_search.best_params_
print("Best hyperparameters found:", best_params)
# Evaluate the best model
best_score = bayes_search.best_score_
print("Best cross-validation score:", best_score)
This example demonstrates Bayesian optimization on a random forest classifier using a synthetic dataset.
The code imports necessary libraries, defines the model and search space, and utilizes the BayesSearchCV
class from scikit-optimize to perform Bayesian optimization. The best hyperparameters are then printed, along with the best cross-validation score.
General Overview of Bayesian Optimization
Unveiling Key Concepts and Principles
Bayesian optimization is a global optimization technique for expensive black-box functions. It’s particularly effective for hyperparameter tuning in machine learning, where evaluating model performance can be computationally expensive.
Two main components drive this approach: the surrogate model and the acquisition function.
- Surrogate model: A probabilistic model, often Gaussian Processes, that approximates the objective function. It captures the uncertainty of the true function, enabling informed decisions about the next point to evaluate.
- Acquisition function: A function that leverages the surrogate model to determine the most promising point in the search space to sample next. Common choices include Expected Improvement, Probability of Improvement, and Upper Confidence Bound.
The interplay between these components allows Bayesian optimization to intelligently balance exploration and exploitation. As a result, model reaches convergence to the optimal solution faster.
Weighing Advantages and Disadvantages
Advantages:
- Efficient search: Bayesian optimization requires fewer evaluations than traditional methods, saving time and computational resources.
- Noise handling: It can handle noisy objective functions, as the surrogate model accounts for uncertainty in the evaluations.
- Flexibility: It’s applicable to a wide range of optimization problems, including those with continuous, discrete, and mixed search spaces.
Disadvantages:
- Complexity: Bayesian optimization can be more complex to implement and understand than simpler techniques, especially for beginners.
- Scalability: The surrogate model’s computational complexity can limit its applicability to high-dimensional problems or those with a large search space.
- Initialization: The method requires an initial set of samples to kick-start the optimization process, which can influence the effectiveness of the search.
Despite these drawbacks, Bayesian optimization remains a powerful tool for hyperparameter tuning. Further, offering significant benefits in terms of search efficiency and adaptability to various optimization challenges.
Bayesian Optimization in XGBoost
Seamless Integration with XGBoost Algorithm
Bayesian optimization can be easily integrated with the XGBoost algorithm to optimize its hyperparameters and improve overall model performance.
XGBoost, short for eXtreme Gradient Boosting, is a popular decision-tree-based ensemble algorithm. Moreover, it has won numerous machine learning competitions due to its speed and accuracy.
To use Bayesian optimization with XGBoost, follow a similar approach as with other machine learning algorithms.
First, define the XGBoost model and specify the hyperparameter search space. Then, use an optimization library like scikit-optimize to perform the Bayesian optimization process.
Illustrating an Example Use Case
Consider a binary classification problem using a synthetic dataset. Let’s optimize the hyperparameters of an XGBoost classifier with Bayesian optimization:
import xgboost as xgb
import numpy as np
from sklearn.datasets import make_classification
from sklearn.model_selection import cross_val_score
from skopt import BayesSearchCV
from skopt.space import Real, Categorical, Integer
# Define the XGBoost model
model = xgb.XGBClassifier()
# Specify the search space for hyperparameters
param_space = {
"learning_rate": Real(0.01, 1.0),
"n_estimators": Integer(50, 500),
"max_depth": Integer(1, 20),
"min_child_weight": Integer(1, 10),
"gamma": Real(0, 1),
"subsample": Real(0.1, 1),
"colsample_bytree": Real(0.1, 1),
}
# Perform Bayesian optimization
bayes_search = BayesSearchCV(
model,
param_space,
n_iter=50,
cv=5,
n_jobs=-1,
scoring="accuracy",
random_state=42,
)
# Load sample dataset and split it into features (X) and target (y)
X, y = make_classification(n_samples=1000, n_features=20, n_classes=2, random_state=42)
# Fit the model with the optimal hyperparameters
bayes_search.fit(X, y)
# Retrieve the best hyperparameters
best_params = bayes_search.best_params_
print("Best hyperparameters found:", best_params)
# Evaluate the best model
best_score = bayes_search.best_score_
print("Best cross-validation score:", best_score)
This example demonstrates how to apply Bayesian optimization to tune an XGBoost classifier’s hyperparameters, leading to improved model performance.
Comparing Optimization Techniques
Bayesian Optimization vs. Grid Search: Speed and Effectiveness
Speed comparison: Bayesian optimization is typically faster than grid search because it requires fewer evaluations.
Grid search exhaustively tests all possible combinations of hyperparameters within a predefined range. While Bayesian optimization intelligently selects the most promising points to evaluate, saving time and computational resources.
Effectiveness: Bayesian optimization often converges to the optimal solution more quickly than grid search, as it leverages a surrogate model to guide the search process.
Grid search might miss the optimal solution if the search space is not finely sampled, requiring a larger number of evaluations for similar accuracy.
Bayesian Optimization vs. Random Search: Advantages and Scenarios
Advantages of Bayesian optimization: Bayesian optimization generally outperforms random search in terms of efficiency and accuracy.
It uses a probabilistic model to guide the search, balancing exploration and exploitation. On the other hand random search evaluates points randomly, with no consideration of previous evaluations.
Appropriate scenarios for each technique:
- Grid search: Works well for low-dimensional problems with a small number of discrete hyperparameters, where an exhaustive search is computationally feasible.
- Random search: Suitable for a quick exploration of the search space, especially when the number of hyperparameters is large. However, it’s less efficient than Bayesian optimization.
- Bayesian optimization: Ideal for expensive black-box functions or when computational resources are limited. Furthermore, it works well for continuous and mixed search spaces and handles noisy evaluations effectively.
In summary, Bayesian optimization stands out as an advanced and efficient method for hyperparameter tuning compared to grid and random search.
However, the choice of technique ultimately depends on the problem’s complexity, the nature of the search space, and the available computational resources.
Common Questions about Bayesian Optimization
Does Google Optimize use Bayesian methods?
Yes, Google Optimize employs Bayesian methods to model the performance of different variations in A/B testing.
By leveraging Bayesian statistics, Google Optimize can provide more accurate estimations of the true conversion rates and make better-informed decisions about which variation performs the best.
When should you use Bayesian optimization?
Bayesian optimization is well-suited for situations where:
- Evaluating the objective function is computationally expensive or time-consuming, such as in hyperparameter tuning of complex machine learning models.
- The search space consists of continuous or mixed variables, as Bayesian optimization can efficiently explore such spaces.
- There’s a need to handle noisy evaluations, as Bayesian optimization’s surrogate model can account for uncertainty in the objective function.
- The problem is black-box, meaning the objective function is not easily differentiable or has unknown properties.
Why is Bayesian optimization considered better than alternatives?
Bayesian optimization offers several advantages over alternative methods like grid search and random search:
- Efficiency: Bayesian optimization requires fewer evaluations to converge to the optimal solution, saving time and computational resources.
- Surrogate modeling: By leveraging a probabilistic model to approximate the objective function, Bayesian optimization can make informed decisions about which points to evaluate next.
- Exploration and exploitation balance: Bayesian optimization balances exploring the search space and exploiting the information gained from previous evaluations, leading to faster convergence.
- Noise handling: Bayesian optimization can effectively handle noisy objective functions, as the surrogate model accounts for uncertainty.
These advantages make Bayesian optimization a preferred choice for many optimization tasks, especially in hyperparameter tuning for machine learning models.
Conclusion
In this article, we have explored the powerful technique of Bayesian optimization for hyperparameter tuning in machine learning.
We’ve discussed its key concepts and principles, compared it to other optimization methods like grid search and random search, and highlighted its advantages and suitable use cases.
We also examined how to implement Bayesian optimization in Python, specifically with the XGBoost algorithm, and addressed common questions about the technique.
Furthermore, we delved into the differences and similarities between MCMC and Bayesian optimization and their respective use cases.
Bayesian optimization is an efficient and versatile optimization technique that can offer significant benefits in terms of search efficiency and adaptability to various optimization challenges.
It’s worth considering incorporating Bayesian optimization into your projects to improve the performance of your models and make the most of your computational resources.
So, don’t hesitate to explore and experiment with Bayesian optimization in your machine learning endeavors. Happy optimizing!